Data Grid - Getting started
Get started with the last React data grid you will need. Install the package, configure the columns, provide rows and you are set.
Installation
Using your favorite package manager, install @mui/x-data-grid-pro
for the full-featured enterprise grid, or @mui/x-data-grid
for the free community version.
// with npm
npm install @mui/x-data-grid@^4.0.0
// with yarn
yarn add @mui/x-data-grid@^4.0.0
The grid has two peer dependencies on Material-UI components. If you are not already using Material-UI in your project, you can install it with:
// with npm
npm install @material-ui/core @material-ui/styles
// with yarn
yarn add @material-ui/core @material-ui/styles
Quick start
First, you have to import the component as below.
To avoid name conflicts the component is named DataGridPro
for the full-featured enterprise grid, and DataGrid
for the free community version.
import { DataGrid } from '@mui/x-data-grid';
Define rows
Rows are key-value pair objects, mapping column names as keys with their values.
You should also provide an id
property on each row to allow delta updates and better performance.
Here is an example
const rows: GridRowsProp = [
{ id: 1, col1: 'Hello', col2: 'World' },
{ id: 2, col1: 'DataGridPro', col2: 'is Awesome' },
{ id: 3, col1: 'Material-UI', col2: 'is Amazing' },
];
Define columns
Comparable to rows, columns are objects defined with a set of attributes of the GridColDef
interface.
They are mapped to rows through their field
property.
const columns: GridColDef[] = [
{ field: 'col1', headerName: 'Column 1', width: 150 },
{ field: 'col2', headerName: 'Column 2', width: 150 },
];
You can import GridColDef
to see all column properties.
Demo
Putting it together, this all you need to get started, as you can see in this live and interactive demo:
import React from 'react';
import { DataGrid, GridRowsProp, GridColDef } from '@mui/x-data-grid';
const rows: GridRowsProp = [
{ id: 1, col1: 'Hello', col2: 'World' },
{ id: 2, col1: 'DataGridPro', col2: 'is Awesome' },
{ id: 3, col1: 'Material-UI', col2: 'is Amazing' },
];
const columns: GridColDef[] = [
{ field: 'col1', headerName: 'Column 1', width: 150 },
{ field: 'col2', headerName: 'Column 2', width: 150 },
];
export default function App() {
return (
<div style={{ height: 300, width: '100%' }}>
<DataGrid rows={rows} columns={columns} />
</div>
);
}
Licenses
The data grid comes with two different licenses:
DataGrid, it's MIT licensed and available on npm as
@mui/x-data-grid
.DataGridPro, it's Commercially licensed and available on npm as
@mui/x-data-grid-pro
. The features only available in the commercial version are suffixed with a icon for the Pro plan or a icon for the Premium plan.You can check the feature comparison for more details. See Pricing for details on purchasing licenses.
Try DataGridPro for free
You are free to install and try DataGridPro
as long as it is not used for development of a feature intended for production.
Please take the component for a test run, no need to contact us.
Invalid license
If you have an enterprise grid running with an expired or missing license key, the grid displays a watermark, and a warning is shown in the console (Material-UI Unlicensed product).
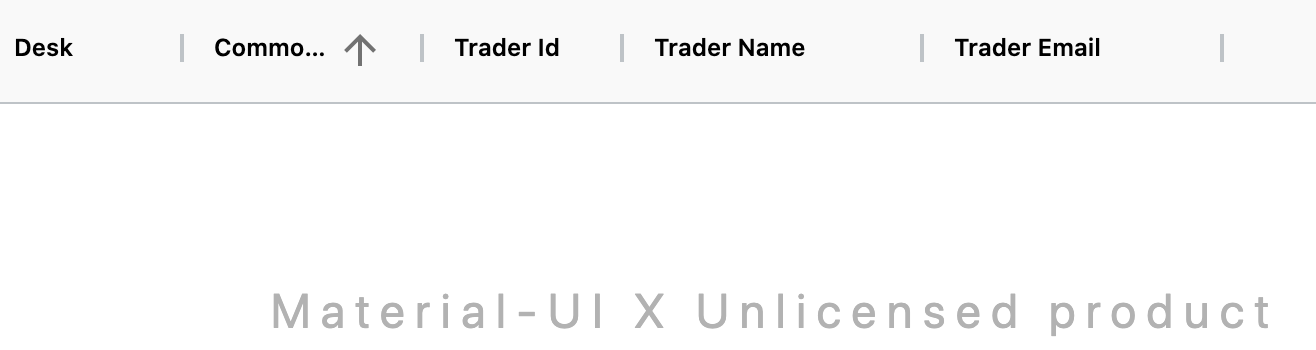
Feature comparison
The following table summarizes the features available in the community DataGrid
and enterprise DataGridPro
components.
All the features of the community version are available in the enterprise one.
The enterprise components come in two plans: Pro and Premium.
Features | Community | Pro | Premium |
---|---|---|---|
Column | |||
Column groups | 🚧 | 🚧 | 🚧 |
Column spanning | 🚧 | 🚧 | 🚧 |
Column resizing | ❌ | ✅ | ✅ |
Column reorder | ❌ | ✅ | ✅ |
Column pinning | ❌ | 🚧 | 🚧 |
Row | |||
Row sorting | ✅ | ✅ | ✅ |
Row height | ✅ | ✅ | ✅ |
Row spanning | 🚧 | 🚧 | 🚧 |
Row reordering | ❌ | 🚧 | 🚧 |
Selection | |||
Row selection | ✅ | ✅ | ✅ |
Multi-row selection | ❌ | ✅ | ✅ |
Range selection | ❌ | ❌ | 🚧 |
Filtering | |||
Quick filter | 🚧 | 🚧 | 🚧 |
Column filters | ✅ | ✅ | ✅ |
Multi-column filtering | ❌ | ✅ | ✅ |
Pagination | |||
Pagination | ✅ | ✅ | ✅ |
Pagination > 100 rows per page | ❌ | ✅ | ✅ |
Editing | |||
Row editing | ✅ | ✅ | ✅ |
Cell editing | ✅ | ✅ | ✅ |
Import & export | |||
CSV export | ✅ | ✅ | ✅ |
🚧 | 🚧 | 🚧 | |
Clipboard | ❌ | 🚧 | 🚧 |
Excel export | ❌ | ❌ | 🚧 |
Rendering | |||
Customizable components | ✅ | ✅ | ✅ |
Column virtualization | ✅ | ✅ | ✅ |
Row virtualization > 100 rows | ❌ | ✅ | ✅ |
Group & Pivot | |||
Tree data | ❌ | 🚧 | 🚧 |
Master detail | ❌ | 🚧 | 🚧 |
Grouping | ❌ | ❌ | 🚧 |
Aggregation | ❌ | ❌ | 🚧 |
Pivoting | ❌ | ❌ | 🚧 |
Misc | |||
Accessibility | ✅ | ✅ | ✅ |
Keyboard navigation | ✅ | ✅ | ✅ |
Localization | ✅ | ✅ | ✅ |
License key installation
Once you purchase a license, you'll receive a license key. This key should be provided to the enterprise package to remove the watermark and the warnings in the console.
import { LicenseInfo } from '@mui/x-data-grid-pro';
LicenseInfo.setLicenseKey(
'x0jTPl0USVkVZV0SsMjM1kDNyADM5cjM2ETPZJVSQhVRsIDN0YTM6IVREJ1T0b9586ef25c9853decfa7709eee27a1e',
);
The grid checks the key without making any network requests.
What's the expiration policy?
The licenses are perpetual, the license key will work forever. However, access to updates is not. If you install a new version of the component for which the license key has expired, you will trigger a watermark and console message. For instance, if you have a one-year license (default), you are not licensed to install a version that is two years in the future.
Support
For crowdsourced technical questions from expert Material-UI devs in our community. Also frequented by the Material-UI core team.
GitHub 
We use GitHub issues exclusively as a bug and feature request tracker. If you think you have found a bug, or have a new feature idea, please start by making sure it hasn't already been reported or fixed. You can search through existing issues and pull requests to see if someone has reported one similar to yours.
StackOverflow 
For crowdsourced technical questions from expert Material-UI devs in our community. Also frequented by the Material-UI core team.
Enterprise support
We provide a private support channel for enterprise customers.
Roadmap
Here is the public roadmap. It's organized by quarter.
⚠️ Disclaimer: We operate in a dynamic environment, and things are subject to change. The information provided is intended to outline the general framework direction, for informational purposes only. We may decide to add or remove new items at any time, depending on our capability to deliver while meeting our quality standards. The development, releases, and timing of any features or functionality remains at the sole discretion of Material-UI. The roadmap does not represent a commitment, obligation, or promise to deliver at any time.